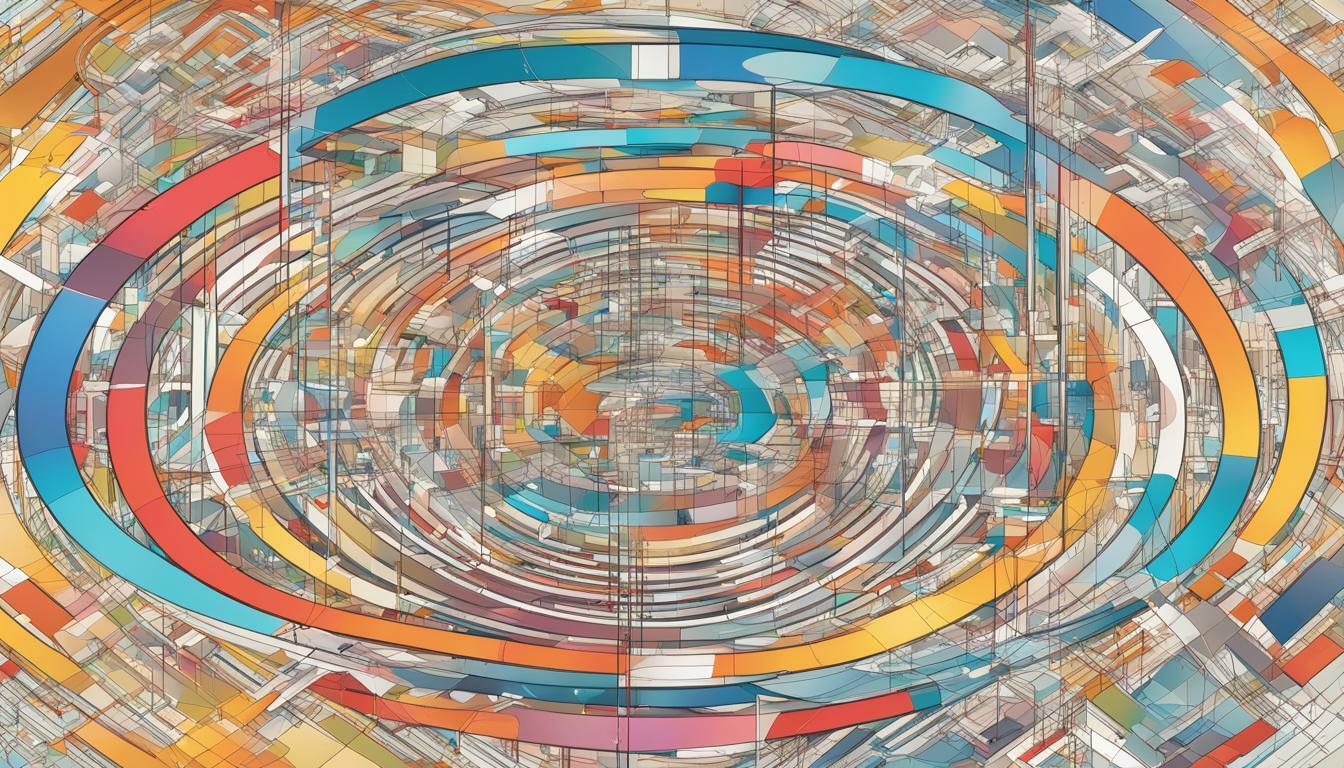
Design patterns are crucial in software development, providing solutions to common programming problems. Using design patterns results in efficient and effective programming, as they offer tested and proven solutions to recurring issues. Software design patterns, design principles, object-oriented design, and software architecture are all part of the design pattern landscape, and mastering them is essential to successful software development.
In this article, we will explore the different types of design patterns, their benefits, and how to implement them effectively.
Key Takeaways:
- Design patterns are crucial in software development
- They offer tested and proven solutions to recurring issues
- Mastering design patterns is essential to successful software development
Understanding Design Patterns
Design patterns are proven solutions to common problems that arise in software development. They have become an essential aspect of software engineering and are widely used in the industry today. Pattern recognition is a crucial skill when it comes to software development, and design patterns allow developers to spot recurring patterns in code and apply them in the best possible way.
Software development can be a complex process, and design patterns provide a framework that helps developers simplify it. Design patterns provide common ground and a shared vocabulary for developers, enabling them to communicate efficiently and avoid misunderstandings. They are based on design principles and best practices that make software development more efficient and effective.
To use design patterns effectively, it is essential to understand the underlying principles that govern their use. Developers who understand design principles and best practices can leverage design patterns to create better software solutions that are more maintainable and scalable.
Pattern Recognition in Software Development
Pattern recognition is a cognitive process that involves the ability to identify similarities or patterns between different objects, situations or events. In software development, this skill is essential for identifying recurring problems or patterns in code and applying design patterns appropriately.
Recognizing patterns can be challenging, but with practice, developers can develop this skill and become more efficient at using design patterns. Design patterns can be applied to a wide range of programming languages and platforms, and mastering pattern recognition can be the key to unlocking their full potential.
Software engineering is an evolving field, and design patterns have become a central aspect of the discipline. They have revolutionized the way software is designed, and developers who understand and apply design patterns well are in high demand.
- Design Patterns
- Pattern Recognition
- Software Development
- Software Engineering
- Design Principles and Best Practices
Types of Design Patterns
Design patterns are divided into three major categories based on their functionality. These categories are known as creational patterns, structural patterns, and behavioral patterns. Each of these categories serves a specific purpose in software development and has its unique set of design patterns to choose from.
Creational Patterns
Creational patterns deal with object creation mechanisms, which increase flexibility and reuse of existing code. These patterns help create objects without directly using the “new” operator and provide a way to instantiate objects based on specific situations.
Creational patterns are further categorized into different patterns like the Factory pattern, which creates objects without specifying the exact type, and the Singleton pattern, which ensures only one instance of the class is created and shared across the application.
Structural Patterns
Structural patterns focus on class composition and object relationships. They provide a clear structure for creating larger, more complex objects and make them easier to understand and manage. Structural patterns are essential in software design as they provide a simple interface for the client to use and provide flexibility in code design.
Adapter pattern and Decorator pattern are examples of structural patterns. Adapter pattern is used when we need to convert the interface of an existing class to the interface the client expects, and Decorator pattern is used to add behavior or functionality to an individual object without changing the class itself.
Behavioral Patterns
Behavioral patterns deal with communication between objects and the assignment of responsibilities between objects. These patterns enable flexibility in communication between objects and provide a way to modify the behavior of objects at runtime.
Observer pattern and Strategy pattern are examples of behavioral patterns. The Observer pattern is used when we need to notify one or more objects when the state of another object changes, and the Strategy pattern is used when we need to select an algorithm for a class based on a specific condition.
Creational Design Patterns
Creational design patterns are concerned with the object instantiation mechanisms, trying to create objects in a manner suitable for the situation. The goal is to provide solutions to instantiate objects in a way that makes code more flexible and reusable.
Factory Pattern
The factory pattern is one of the most widely used creational design patterns. It provides a way to create objects without specifying the exact class of the object that will be created. The factory pattern uses a creator class and a set of concrete classes to accomplish this. The creator class is responsible for instantiating the concrete classes. This pattern promotes loose coupling between objects, making it easier to modify or extend the system. It is an ideal choice when the object creation logic involves some complexity or when we have a large number of objects to create.
Singleton Pattern
The singleton pattern is another popular creational design pattern that is used to ensure that a class has only one instance while providing a global point of access to that instance. This pattern is commonly used in situations where a single object is needed to coordinate actions across a system. It allows for the creation of a single instance of a class that can be used throughout the system, promoting code reuse and reducing memory usage. However, it is essential to ensure that thread safety is maintained when implementing the Singleton pattern.
Structural Design Patterns
Structural design patterns focus on class composition and object relationships. They help to simplify the design of complex systems by identifying simple ways to compose classes and objects. Two common structural design patterns are the adapter pattern and the decorator pattern.
Adapter Pattern
The adapter pattern allows two incompatible interfaces to work together by wrapping an object with a different interface. This pattern is useful when you need to integrate code from external libraries or systems that use a different interface from your own code. The adapter pattern makes it easy to create adapters that can communicate between the two systems, without having to rewrite or modify the existing code.
Pros | Cons |
---|---|
Allows integration of incompatible systems. | Can add extra complexity to the system. |
Simplifies code reuse by creating a standard interface. | May impact performance due to additional layers of abstraction. |
Decorator Pattern
The decorator pattern allows you to add functionality to an object dynamically, without changing its original structure. This pattern is useful when you need to add new features to an object at runtime. The decorator pattern uses a wrapping approach similar to the adapter pattern, but with the added benefit of being able to add multiple decorators to an object.
Pros | Cons |
---|---|
Provides a flexible way to add new functionality to objects. | Can lead to a large number of small classes if used excessively. |
Allows objects to have functionality added at runtime. | May impact performance due to additional layers of abstraction. |
Structural design patterns are valuable tools for simplifying complex designs and promoting code reuse and maintainability. The adapter pattern and decorator pattern are just two examples of the many structural design patterns available to software developers.
Behavioral Design Patterns
In software development, behavioral patterns are used to determine how objects interact with one another and how responsibilities are assigned. Design patterns that fall under this category are used to manage communication between objects and make it easier to modify the behavior of an object.
The observer pattern is a common example of a behavioral pattern, which allows objects to observe and react to changes in another object’s state. It involves an observer object that keeps track of any changes to the observed object and updates itself and other dependent objects accordingly.
The strategy pattern is another example of a behavioral pattern that lets you choose between different algorithms or strategies based on specific conditions. In this pattern, different strategies are encapsulated and can be swapped out at runtime, making it easier to modify an object’s behavior without changing its class.
Both these patterns are useful in managing the communication between objects and assigning responsibilities, making software development more efficient and effective.
Implementing Design Patterns
Implementing design patterns in software development can greatly improve code quality and efficiency. One major benefit of design patterns is their contribution to code reusability, allowing for the creation of more scalable and maintainable software.
However, it is important to follow best practices when implementing design patterns to ensure their effectiveness. One key aspect is to understand the specific problem that a design pattern is intended to solve before applying it. This helps prevent unnecessary complexity and ensures that the pattern is truly the best solution for the given problem.
Another best practice is to prioritize readability and maintainability when implementing design patterns. Clear and well-organized code makes it easier for other developers to understand and modify the code in the future.
Additionally, it is important to consider the extensibility of the code when incorporating design patterns. This allows for future adaptations and feature additions without requiring major rewrites or restructuring of the codebase.
Incorporating design patterns into the software engineering process can greatly enhance the overall quality and efficiency of software development. By prioritizing code reusability, best practices, and thoughtful implementation, developers can ensure the successful integration of design patterns into their projects.
Design Patterns and Software Architecture
Design patterns play a crucial role in software architecture, providing a foundation for building scalable applications and maintaining clean, manageable code. Effective software architecture requires a deep understanding of design patterns and how they can be applied to solve common problems in software development.
By implementing design patterns, developers can create modular and reusable components that can be easily integrated into larger software systems. This not only saves time and resources, but also ensures that software architecture remains flexible and adaptable to changing requirements.
Scalability is a critical factor in software architecture, and design patterns help ensure that software systems can handle increasing loads and complexity. By following best practices for software architecture and integrating design patterns, developers can create software systems that are more resilient, efficient, and scalable.
Another important aspect of software architecture is maintainability. By using design patterns, developers can create code that is easier to update and modify, reducing the risk of introducing bugs and errors. This helps ensure that software systems remain reliable and stable over time.
Ultimately, design patterns are an essential tool for any software architect, providing a framework for building scalable, maintainable, and extensible software systems.
Design Patterns in Object-Oriented Design
Design patterns play a crucial role in object-oriented design, a programming approach that emphasizes the use of objects to represent real-world entities and concepts. Encapsulation, inheritance, and polymorphism are fundamental concepts in object-oriented design, and design patterns provide effective ways to incorporate these principles into software development.
Encapsulation involves creating classes that contain both data and methods to manipulate that data, encapsulating them into a single unit. Design patterns like the Factory Method Pattern and the Singleton Pattern can be used to implement encapsulation effectively.
Inheritance involves the creation of subclasses that inherit attributes and methods from a parent class. Design patterns like the Template Method Pattern and the Strategy Pattern can be used to implement inheritance effectively.
Polymorphism involves the ability of objects to take on multiple forms. Design patterns like the Observer Pattern and the Iterator Pattern can be used to implement polymorphism effectively.
By incorporating design patterns into object-oriented design, developers can create robust and flexible software that can adapt to changing requirements and use cases. Effective use of design patterns can also improve code readability, maintainability, and extensibility, making it easier to debug and update software over time.
Design Patterns Best Practices
Design patterns can greatly enhance the quality and maintainability of software projects. However, in order to use them effectively, it is important to follow best practices that ensure code readability, maintainability, and extensibility.
Code readability is essential for ensuring that code can be easily understood and modified in the future. When using design patterns, it is important to use descriptive names for patterns and classes, as well as clear and concise comments. This will help other developers understand the purpose of the code and how to modify it if necessary.
Maintainability is key to ensuring that software can be easily modified and updated over time. When using design patterns, it is important to avoid complex code and to keep code as modular as possible. This will make it easier to modify individual components of the software without affecting other parts of the code.
Extensibility is critical for ensuring that software can be easily extended to meet new requirements. When using design patterns, it is important to anticipate future changes and to design code that can be easily extended without breaking existing functionality. This can be achieved by using interfaces and abstract classes to define the behavior of components, and by using composition rather than inheritance to create relationships between objects.
In addition to these best practices, it is important to follow established design principles and patterns when implementing design patterns. This will help ensure that code is consistent, predictable, and maintainable over time.
Conclusion
Design patterns play a crucial role in software development, providing a structured approach to software design that leads to efficient and effective programming. By understanding and implementing design patterns, developers can build scalable applications with maintainable code, while adhering to best practices and maximizing code reusability.
Whether it’s creational, structural, or behavioral design patterns, each type offers unique benefits and can be applied to a variety of use cases. The observer pattern, factory pattern, strategy pattern, adapter pattern, and decorator pattern are some of the most commonly used examples.
When designing software architecture, design patterns are a key consideration in achieving scalable and maintainable solutions. By focusing on concepts like encapsulation, inheritance, and polymorphism, developers can create robust and flexible object-oriented systems that can adapt to changing requirements.
To ensure effective use of design patterns, it’s important to adhere to best practices that promote code readability, maintainability, and extensibility. With these practices in mind, developers can take full advantage of design patterns to create high-quality, efficient, and effective software.
Overall, mastering design patterns is essential for any developer looking to take their programming skills to the next level. By understanding and implementing these patterns effectively, developers can create software that meets the highest standards of quality and performance for their users.
FAQ
Q: What are design patterns?
A: Design patterns are reusable solutions to common software design problems. They provide a way to solve problems efficiently and effectively by leveraging established best practices.
Q: Why are design patterns important in programming?
A: Design patterns play a crucial role in programming by promoting code reusability, making code more maintainable, and improving overall software design. They help developers solve common programming problems and create efficient and effective solutions.
Q: What are the different types of design patterns?
A: Design patterns can be categorized into three main types: creational patterns, structural patterns, and behavioral patterns. Each type focuses on addressing different aspects of software design and development.
Q: Can you provide examples of creational design patterns?
A: Two common examples of creational design patterns are the factory pattern and the singleton pattern. The factory pattern provides a way to create objects without specifying their exact class, while the singleton pattern ensures that only one instance of a class is created.
Q: What are structural design patterns?
A: Structural design patterns deal with class composition and object relationships. Examples of structural design patterns include the adapter pattern, which allows objects with incompatible interfaces to work together, and the decorator pattern, which adds additional behaviors to an object dynamically.
Q: What are behavioral design patterns used for?
A: Behavioral design patterns are used to manage communication between objects and the assignment of responsibilities. Two common examples are the observer pattern, which allows objects to be notified of changes in the state of other objects, and the strategy pattern, which enables selecting an algorithm at runtime.
Q: How can design patterns be implemented in software development?
A: Design patterns can be implemented by following best practices and integrating them into the software engineering process. It is important to consider code reusability and follow established design principles to ensure effective implementation of design patterns.
Q: What is the relationship between design patterns and software architecture?
A: Design patterns contribute to the development of scalable and maintainable software architecture. They provide solutions to common architectural problems and help create software systems that are flexible and adaptable.
Q: How do design patterns relate to object-oriented design?
A: Design patterns are closely related to object-oriented design principles such as encapsulation, inheritance, and polymorphism. They enhance the capabilities and flexibility of object-oriented systems by providing proven solutions to design problems.
Q: What are some best practices for using design patterns?
A: When using design patterns, it is important to prioritize code readability, maintainability, and extensibility. Following established best practices and guidelines ensures that design patterns are implemented effectively and contribute to efficient software development.